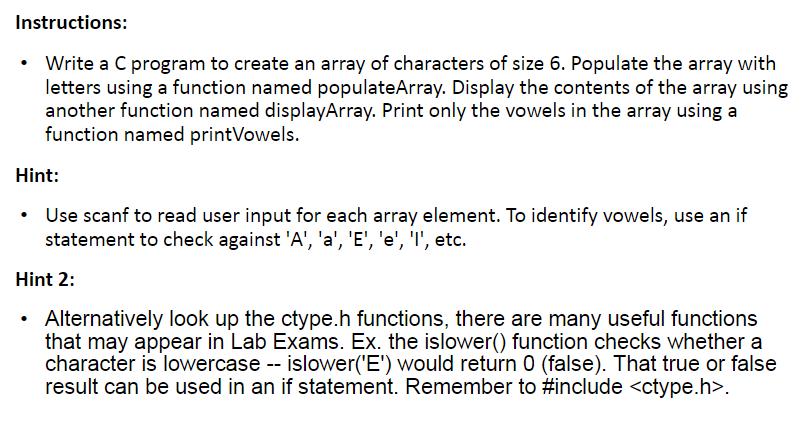
Instructions: – Write a C program to create an array of characters of size 6. – Populate the array with letters using a function named populateArray. – Display the contents of the array using another function named displayArray. – Print only the vowels in the array using a function named printVowels. Hint: – Use scanf to read user input for each array element. – To identify vowels, use an if statement to check against ‘A’, ‘a’, ‘E’, ‘e’, ‘I’, etc. – Alternatively, look up the ctype.h func