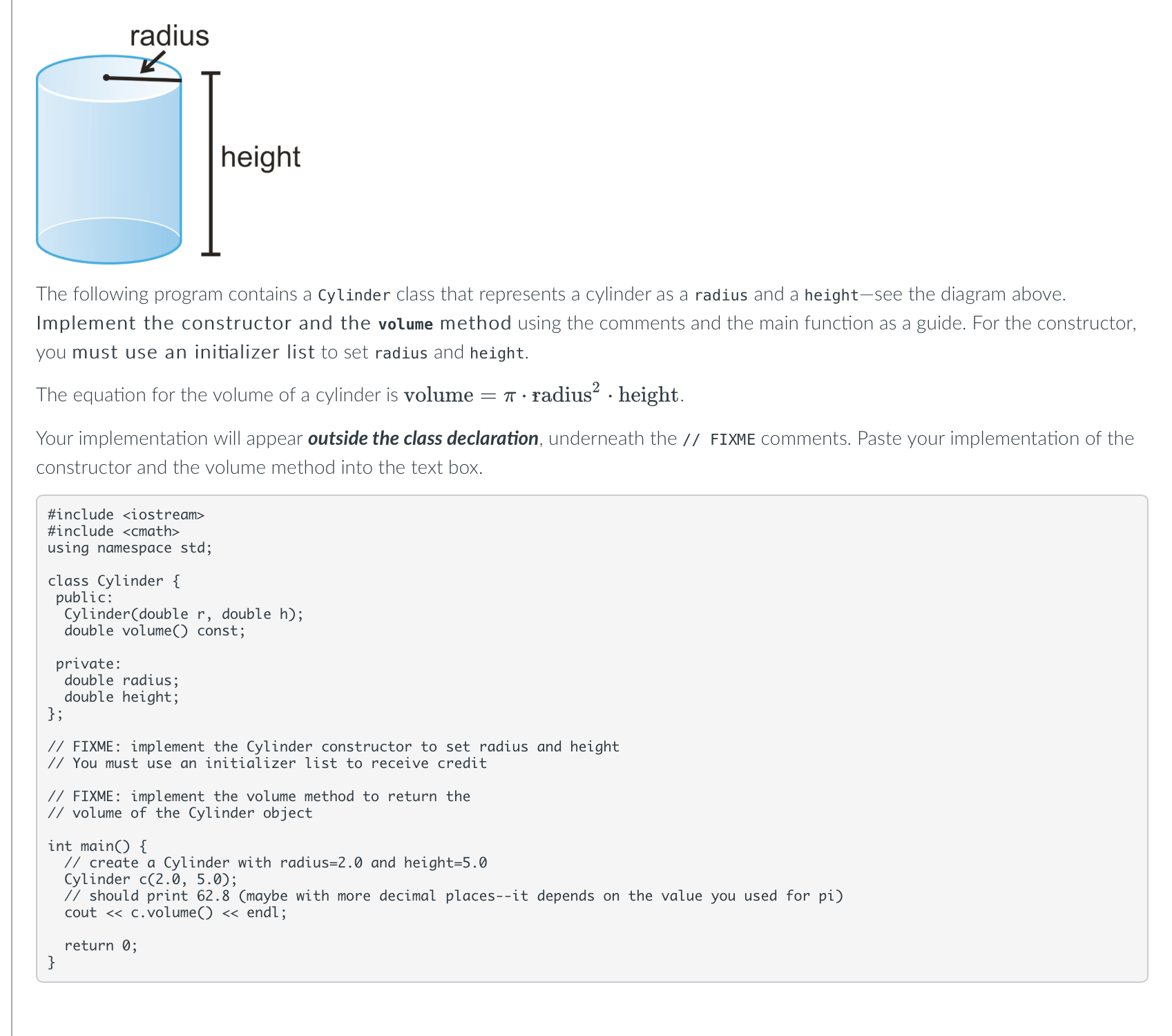
The following program contains a Cylinder class that represents a cylinder as a radius and a height – see the diagram above. Implement the constructor and the volume method using the comments and the main function as a guide. For the constructor, you must use an initializer list to set the radius and height. The equation for the volume of a cylinder is volume = radius^2 * height. Your implementation will appear outside the class declaration, underneath the // FIXME comments.
“`
#include
#include
using namespace std;
class Cylinder {
public:
Cylinder(double r, double h);
double volume() const;
private:
double radius;
double height;
};
// FIXME: implement the Cylinder constructor to set radius and height
// You must use an initializer list to receive credit
// FIXME: implement the volume method to return the volume of the Cylinder object
int main() {
// create a Cylinder with radius = 2.0 and height = 5.0
Cylinder c(2.0, 5.0);
// should print 62.8 (maybe with more decimal places – it depends on the value you used for pi)
cout << c.volume() << endl;
return 0;
}
```
