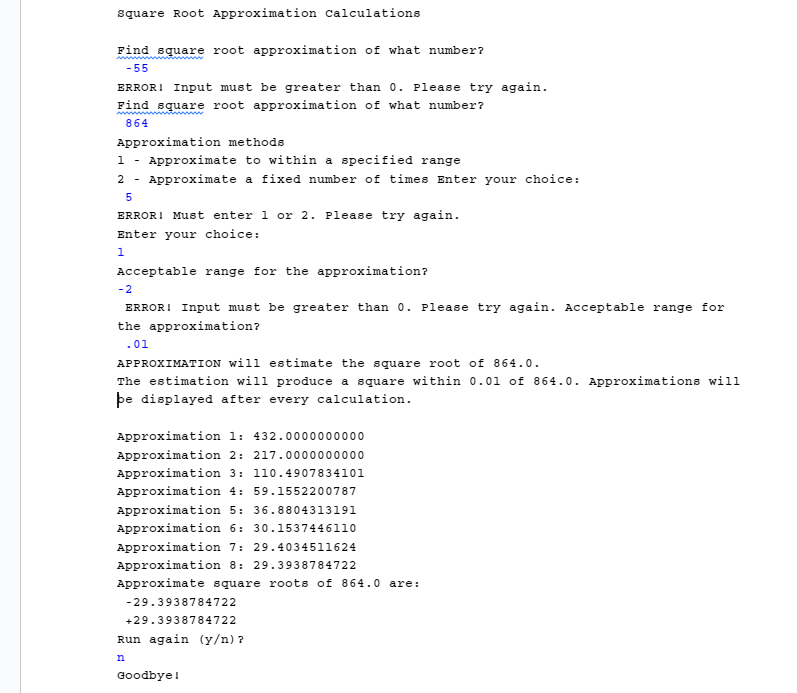
IN JAVA PLEASE HELP IM SO CONFUSED I THINK I GOT METHOD ONE DOWN BUT THE REST IS so confusing You are not allowed to use break or return statements to exit from any loop. So all loops must exit only via their test condition evaluating to false.Things to notice about this formula: num will always be the same ππππΉππππ§ is the previous value that was calculated ππππΉππππ§+π is the current value being calculated For example, the first four square root approximation values that would be calculated f