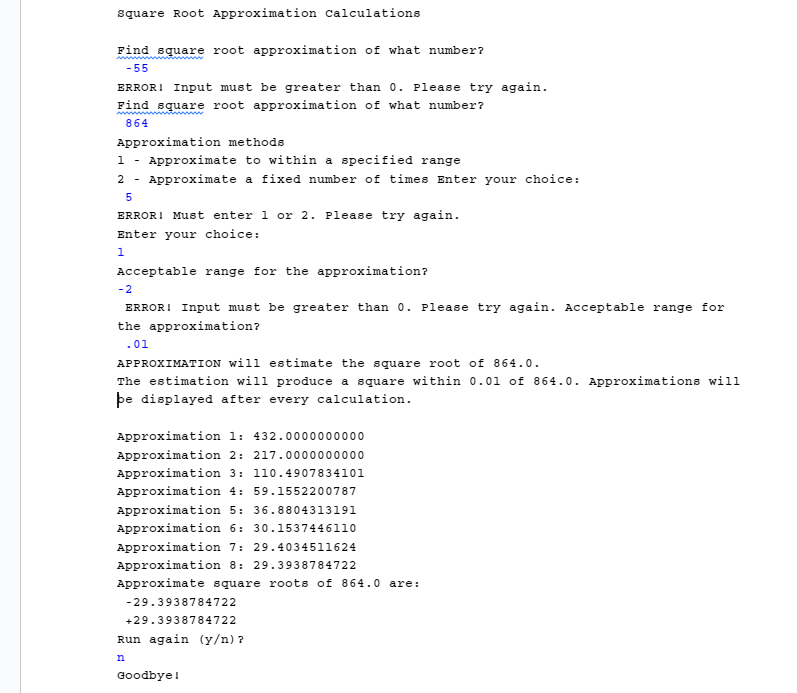
IN JAVA PLEASE HELP IM SO CONFUSED I THINK I GOT METHOD ONE DOWN BUT THE REST IS so confusing You are not allowed to use break or return statements to exit from any loop. So all loops must exit only via their test condition evaluating to false.Things to notice about this formula: num will always be the same ππππΉππππ§ is the previous value that was calculated ππππΉππππ§+π is the current value being calculated For example, the first four square root approximation values that would be calculated for the number 36: ππππΉππππ = 36 / 2.0 = 18.0 ππππΉππππ = (18.0 + 36.0/18.0) / 2.0 = 20.0 / 2.0 = 10.0 ππππΉππππ = (10.0 + 36.0/10.0) / 2.0 = 13.6 / 2.0 = 6.8 ππππΉππππ = (6.8 + 36.0/6.8) / 2.0 = 12.09411765 / 2.0 = 6.047058824 As you can see, even after just 4 repetitions, the result is getting pretty close to 6! You will write a program to approximate the square root of a number, using this formula, and applying it two different ways with user input error checking. NOTE: You cannot use the built-in Java Math.sqrt(x) method anywhere in your code.At least one boolean variableβs value to make a decision (where appropriate) At least one while loop (where appropriate) At least one do-while loop (where appropriate) At least one for loop (where appropriate)First write a method definition for method 1, placing the code after the definition for the main() method. Method 1 will read, validate, and return a floating point (double) value entered by the user, ensuring that it is positive and non-zero. The method will:Have a descriptive method name, including a verb that describes its actions Have two parameters: o a Scanner to read input from the keyboardExample: public static returnType someMethod (Scanner scnr) When this method is called, the argument will be the main() method Scanner object a String description, describing what is being read (to be used as the prompt) Until the user enters valid input (i.e. a positive and non-zero number), use the String parameter to prompt for and read the input , issuing this error message for invalid inputs: ERROR! Input must be greater than 0. Please try again.Return a valid positive and non-zero double value.Define necessary variables, including a Scanner variable to read input from the keyboard. Display this program description, followed by a blank line: Square Root Approximation Calculations Call method 1 to read and save the number whose square root will be approximated, using the following, as the prompt argument: Find square root approximation of what number? Next, write the method definition for method 2, named calcApproxSqrRoot(). Place the code after the definition for the method 1. The method will continuously apply the formula from page 1, until the calculation is within a certain acceptable range.Keep calculating a new sqrRoot approximation until multiplying the approximation by itself results in a number that is within the acceptable range of the original number. Using the example of finding the approximate square root of 36, from page 1, suppose the acceptable range was 1.0: The third approximation is 6.8 6.8 x 6.8 = 46.24, which is NOT within the acceptable range of 1.0 of 36. So continue. The fourth approximation is 6.047058824 6.047058824 x 6.047058824 = 36.56692042, which is within 1.0 of 36. So stop. HINT: You can use Javaβs built-in absolute value method, e.g., Math.abs()Have two double parameters: the double number whose square root will be approximated the double acceptable range Display one blank line, and then display the values the method will use, as follows, (do not use printf(), because we do not want to format the numeric values in this output):APPROXIMATION will estimate the square root of 36.0. The estimation will produce a square within 0.1 of 36.0. Approximations will be displayed after every calculation.At the top of the method, define a local double type variable to hold the square root approximation. Remember to also define any other local variables needed at the top of the method. Initialize your variable to the value for ππππΉππππ and display one blank line followed by the value rounded to 10 decimal places, as shown belowApproximation 1: 18.0000000000Within a loop, until the square root approximation is within the acceptable range (hint: multiply the approximation by itself to see how close it is the actual squared value) o Apply the formula to calculate a more accurate value for the square root approximation o Display the calculated square root approximation to 10 decimal placesApproximation 1: 18.0000000000 Approximation 2: 10.0000000000 Approximation 3: 6.8000000000 Approximation 4: 6.0470588235 Approximation 5: 6.0001831083After the loop exits: o Return the final value of the square root approximation, from the last calculationFirst display a blank line. Determine which technique to use for calculating the approximation, using this menu and prompt:Approximation methods 1 – Approximate to within a specified range 2 – Approximate a fixed number of times Enter your choice: Add a decision statement to call the correct method When 1 is chosen: o Call method 1 a second time to read and save the acceptable range for the approximation, using the following, as the prompt argument: Acceptable range for the approximation? NOTE: Calling the same method again works because both user inputs should be positive and non-zero. The only difference between the two calls will be the prompt that is passed in as the argument for the second parameter, and what variable will be used to store the returned value. o Call method 2, supplying the needed arguments in the call. When 2 is chosen: o FOR NOW, do nothing Display another blank line, followed by the result returned from the method call, as shown below. o Do not use printf() for the FIRST line (do not want to format the number) o Format the positive and negative results rounded to 10 decimal places, using a width of 20 and the printf() + flag that causes signs to show. Example for square root of 36 with an acceptable range of 0.1:Approximate square roots of 36.0 are: -6.0001831083+6.0001831083 Next, write the method definition for method 3, an overloaded method with the same name, calcApproxSqrRoot(). Place the code after the definition for the method 2. This method will apply the formula from page 1 a fixed number of times, displaying approximation values at specified intervals. So it will display the value of every Xth square root approximation, with the value of X specified by the user.For example, if the user wants to display every 3rd square root approximation, then the program should display the results after the 3rd, 6th, 9th, etc. square root approximation has been calculatedAlthough overloaded methods have the same method name, they must have different parameters (parameter types, or the number of parameters). Therefore, this method will: Have three parameters, one double and two integers: o the double number whose square root will be approximated o the integer number of times to apply the approximation formula o the integer display intervalDisplay one blank line, and then display the values the method will use, as follows: APPROXIMATION will estimate the square root of 11111.1, by applying the approximation formula 7 times. Approximations will be displayed after every 3 calculation(s).Again, at the top of the method, define a local double type variable to hold the approximate value of the square root, and initialize it to the value for ππππΉππππ o Remember to also define any other local variables needed at the top of the method.Display one blank line. When required by the display interval, display the first approximation rounded to 10 decimal places. Within a loop, until the approximation has been calculated the correct number of times: o Apply the formula to calculate a more accurate value for the square root approximation o If the calculation falls on a display interval, display the approximation count and the square root approximation to 10 decimal places. After the loop exits return the final value of the square root approximation, from the last calculation (even if it was not displayed). Add the following code to your decision statement action for when 2 is chosen o Call method 1 a third time to read the number of times to apply the approximation formula. Pass in the following, as the prompt argument: Number of times to apply the approximation formula?Convert and save the returned value as an integer. o Call method 1 a fourth time to read the display interval. Pass in the following, as the prompt argument: Display approximation after every how many calculations? Again, convert and save the returned value as an integer. o Call method 3, supplying the needed arguments in the call.Approximation 3: 1391.3867802584Approximation 6: 194.4192820682 lines displayed by calcApproxSqrRoot()Approximate square roots of 11111.1 are: lines displayed by main() -125.7847388377 +125.7847388377Add code to error check that the approximation method chosen is valid. Modify the code in the main() method as follows: Add a loop around the code that prompts for the choice and reads the approximation method that repeats until the user input is valid (do not repeat the menu, just the choice prompt). o When the input is not valid, issue the error message. ERROR! Must enter 1 or 2. Please try again. Modify the main() method code to repeat the program, as follows: Display a blank line, and then ask if the user wants to run the program again with the prompt: Run again (y/n)? As long as the user enters βyβ or βYβ to the question, display 2 blank lines and then loop to run the program again.Be sure to insert the loop so that the previously written code is re-used when the program repeats, and your code does not have duplications. When the user chooses not to run the program again, display: Goodbye!
