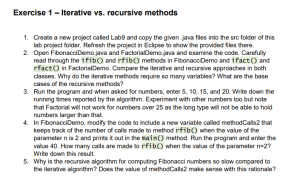
Exercise 1 – Iterative vs. recursive methods 1. Create a new project called Lab9 and copy the given .java files into the src folder of this lab project folder. Refresh the project in Eclipse to show the provided files there. 2. Open FibonacciDemo.java and FactorialDemo.java and examine the code. Carefully read through the ifib() and rfib() methods in FibonacciDemo and ifact() and rfact() in FactorialDemo. Compare the iterative and recursive approaches in both classes. Why do the iterative method