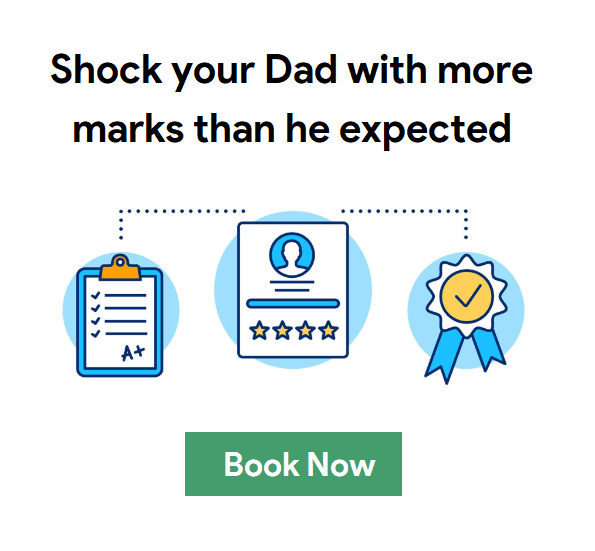
Write this in Python: The goal of this project is to practice your Application and Transport Layer skills by implementing (i) a cache service for file exchange, and (ii) two reliable data transport protocols. The project will be completed in two phases. In the first phase, all students will implement two versions of the program: one that uses stock TCP1 for reliable data transfer; and one that implements stop-and-wait (SNW) reliability at the application layer and uses UDP for transport. In the second phase, graduate students will be asked to evaluate and compare the stock TCP and the stop-and-wait implementations using Wireshark. Your application will support file upload and download using a cache. There will be three components to your program: a client, a server, and a cache. All exchanges (upload or download) will be initiated by the client. Each attempt to download a file will first check with a cache to see if the file is available locally, and only if a target file is unavailable locally, it will be requested from the server. Each attempt to upload a file will store that file directly onto the server, not including the cache in the communication process. • Supported commands: Your program should allow a user to upload and download files, and to quit the program. To this end, you will implement the following commands: • Upload: Copy a file from the client to the server using the put command, which takes as an input argument the full path to a file on the client. Upon a successful receipt of a file, the origin server would send back “File successfully uploaded.” message and close the connection. This functionality should follow the Upload interactions illustrated in Figure 1. Example execution with prompts on the client: put File successfully uploaded. • Download: Copy a file from the server to the client using the get command, which also takes as an argument the full path to a file on the server. This functionality should follow the Download interactions. Specifically, the client should first connect to the cache to download the file locally. Upon successful receipt of the file from the cache, the client would close the connection and display “File delivered from cache.”. Note that this message should come from the cache, rather than being generated locally on the client. If the cache determines that the file is unavailable locally, the cache will request the file from the origin server on behalf of the client, store the file locally for further requests and deliver the file to the client. A control message sent from the cache to the client stating “File delivered from origin.” must be displayed to the user. Example execution with prompts on the client: – File available on cache: get
