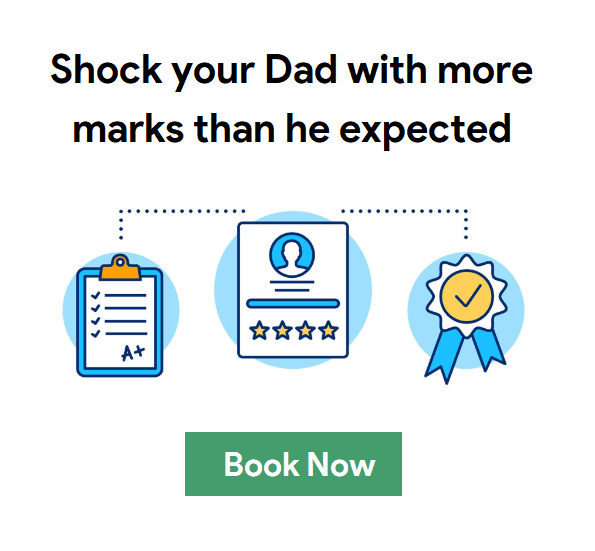
Using C++, implement the Person/Student/Employee/Instructor/Administrator hierarchy of classes that we used for an example of inheritance at the beginning of the course. There will also be a new class Course that describes a course that a student can take or an instructor can teach. All of these classes will descend from the abstract class A2ListItem, which has the following abstract behaviours:virtual int get_id() returns an integer that is used to search or order a sorted list of objectsvirtual void print() prints the contents of the object (short form. i.e basic information from the class)virtual void print_all() prints the contents of the object (long form i.e. additional information such as list of registered students, list of courses registered, etc).You will need to meaningfully override these (and possibly the destructor) in your subclasses. You must implement all six of the classes listed above, plus any other classes you require. How you organize and distribute work in the hierarchy is up to you, though your classes must have the following data and behaviours:Person and all its subclasses have a number (employee/student ID) and a name (string)Course has a number and name, and a list of all of the students who are registered in the courseStudent has a list of all the course number the student is registered in; these do not need to refer to a course objectEmployee has a method weekly_pay() (which can be pure virtual) that calculates and returns that employee’s weekly payInstructor has a list of all the courses the instructor teaches; these should refer back to the corresponding course object. Instructors also have an annual salary, and their weekly pay = salary / 52Administrator has an hourly wage and a number of hours worked per week, and their weekly pay = hourly wage * hours per weekThe data for your program comes from an input file which has values separated by commas, as follows:course,2140,Data Structures and Algorithms
course,2160,Programming Practices
student,2010,Elyse Little,2160,2140
instructor,3030,Cataleya McIntyre,35000,2140,2160
administrator,3039,Cian Gardner,18,40A course has a number and name. A student has a number and name, and then the numbers of all the courses they are registered in. An instructor has a number and name, an annual salary, and the numbers of all the courses they teach. An administrator has a number and name, hourly wage, and hours worked per week.Courses are listed before the students who are registered in them and the instructors who teach them. If you find an invalid course number (or one that has not yet been read in), print an error message, do not add it to the student or instructor, but create the object with the available data. There will not be any errors in the format of the input file. It is possible for a student or instructor to not have any courses.In addition to the A2ListItem class you are given an abstract A2List class that can be used to store lists of items. It has the following methods that you can use:void add(A2ListItem *value) adds the value to the listA2ListItem *find_by_key(int key) returns the list item whose id matches key, or nullptr if no matching item was foundvoid print() calls print() on every item in the listvoid print_all() calls print_all() on every item in the listvoid destroy_contents() (protected) deletes all of the items in the lista virtual destructor that you will need to overrideYou will need to write two subclasses of A2List, one that calls destroy_contents() from the destructor and one that does not. In the end you should have destroyed all of the objects that were created; the final line of output should say that zero objects were leaked.These classes, and a sample main() program that demonstrates how file input works in C++, are posted as a2q2.cpp. Rename this file appropriately and add your own code to it. You can modify all of the given main program except for the output showing the count of leaked objects. Your main program should still accept the name of an input file as a command line parameter.Following is the starting code:#include
All Study Co-Pilots are evaluated by Gotit Pro as an expert in their subject area.