Write the entire code for the following: The purpose of this assignment is to learn use of the simplest type of functions.Problem Statement#include
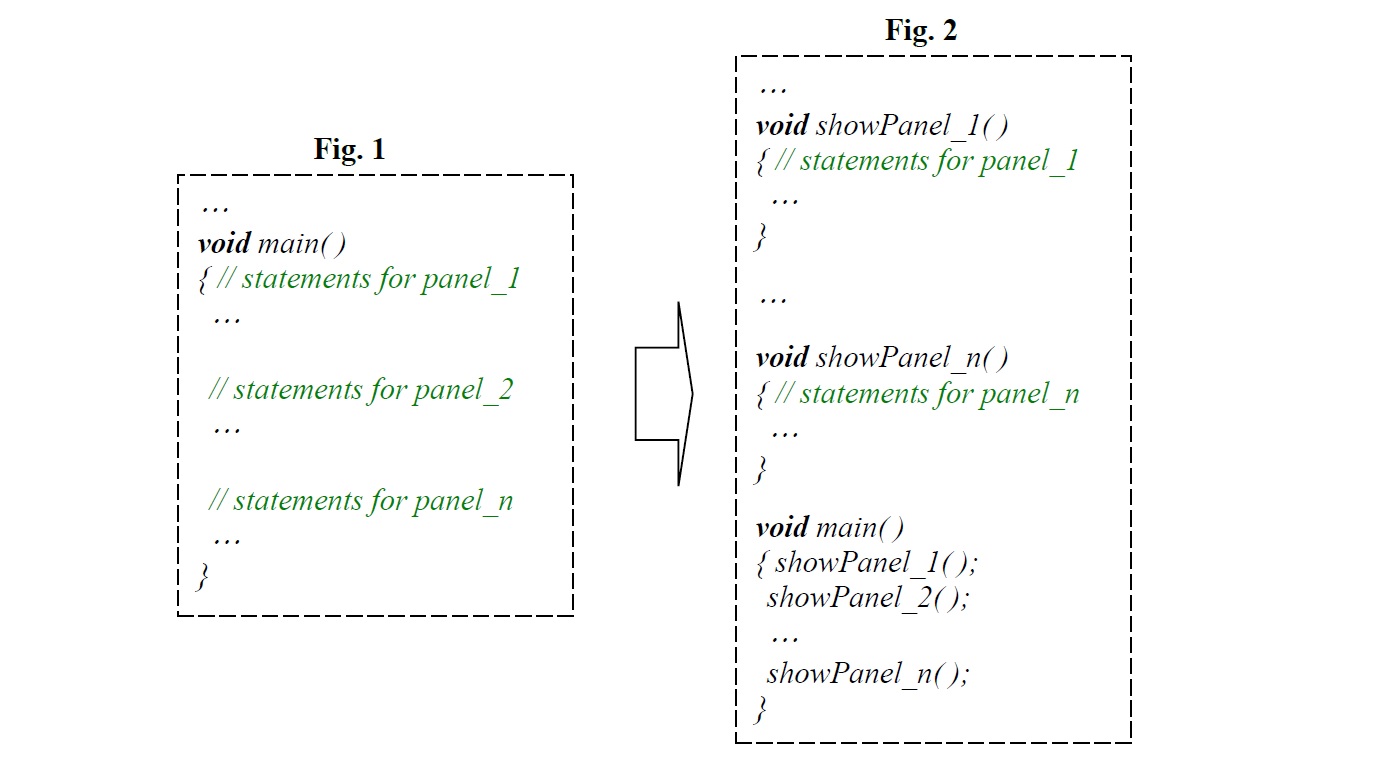
Flash sale unlocked 25% off with code “SUMMER”
Write the entire code for the following: The purpose of this assignment is to learn use of the simplest type of functions.Problem Statement#include
Meditation williamsburg kogi blog bushwick pitchfork polaroid austin dreamcatcher narwhal taxidermy tofu gentrify aesthetic.
Humblebrag ramps knausgaard celiac, trust fund mustache. Ennui man braid lyft synth direct trade.
On all orders above $50
30 days money back guarantee
Offered in the country of usage
PayPal / MasterCard / Visa