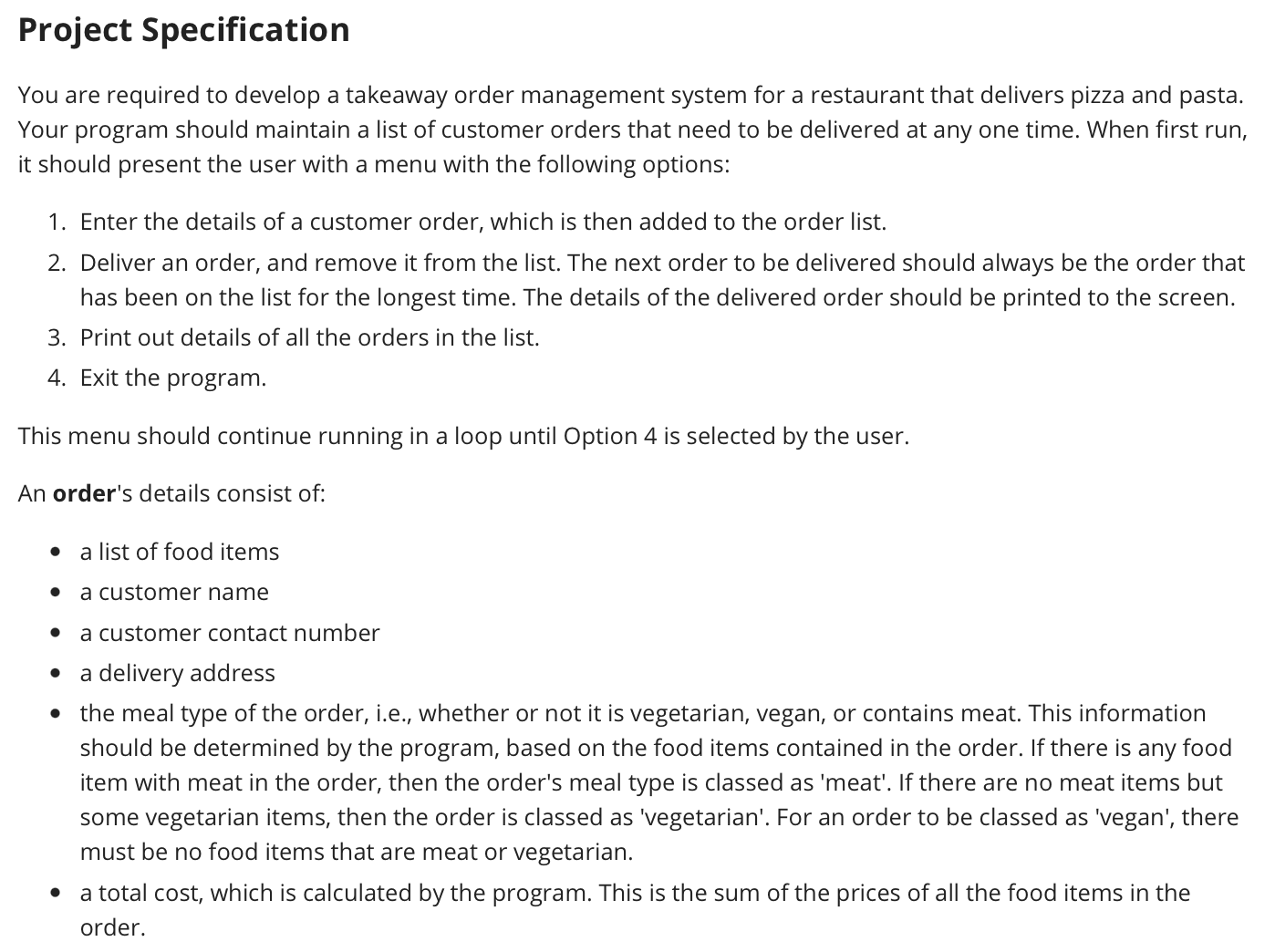
You are required to develop a takeaway order management system for a restaurant that delivers pizza and pasta. Your program should maintain a list of customer orders that need to be delivered at any one time. When first run, it should present the user with a menu with the following options: 1. Enter the details of a customer order, which is then added to the order list. 2. Deliver an order, and remove it from the list. The next order to be delivered should always be the order that has been on