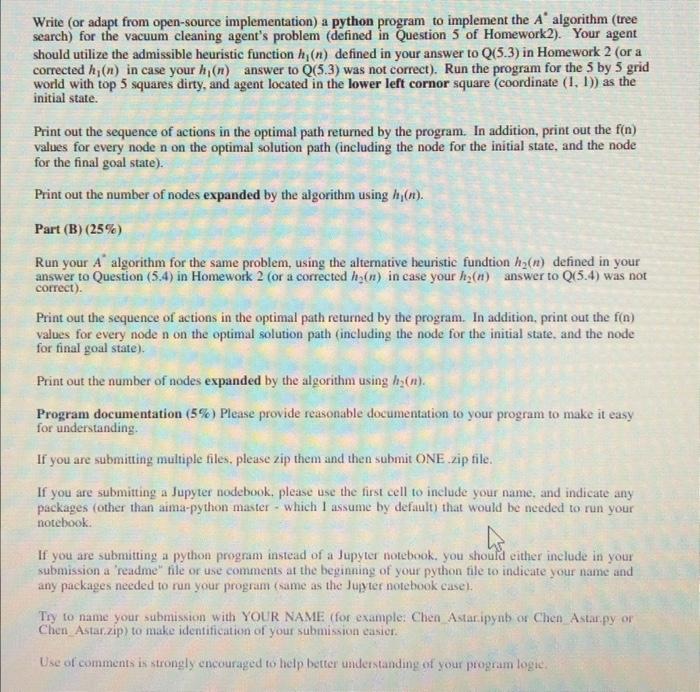
Here we have a vacuum cleaning agent that can sense the environment and perform actions to move around and vacuum clean dirty squares. We assume a 5 by 5 grid world known to the agent. The environment is fully observable: the percepts give complete information about the Dirty/Clean status of every square and the agent’s location. The environment is deterministic: A clean square remains clean and a dirty square remains dirty unless the agent cleans it up. The actions available for the agent are: