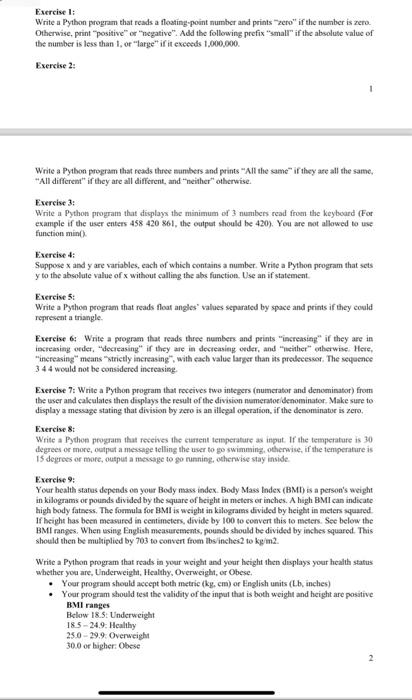
Exercise 1:
Write a Python program that reads a floating-point number and prints “zero” if the number is zero. Otherwise, print “positive” or “negative”. Add the following prefix “small” if the absolute value of the number is less than 1, or “large” if it exceeds 1,000,000.
Exercise 2:
Write a Python program that reads three numbers and prints “All the same” if they are all the same, “All different” if they are all different, and “neither” otherwise.
Exercise 3:
Write a Python program that displays the minimum of 3 numbers read from the keyboard. For example, if the user enters 458, 420, and 61, the output should be 420. You are not allowed to use the function min().
Exercise 4:
Suppose x and y are variables, each of which contains a number. Write a Python program that sets y to the absolute value of x without calling the abs() function. Use an if statement.
Exercise 5:
Write a Python program that reads four angles values separated by spaces and prints if they could represent a triangle.
Exercise 6:
Write a program that reads three numbers and prints “increasing” if they are in increasing order, “decreasing” if they are in decreasing order, and “neither” otherwise. Here, “increasing” means “strictly increasing”, with each value larger than its predecessor. The sequence 344 would not be considered increasing.
Exercise 7:
Write a Python program that receives two integers (numerator and denominator) from the user and calculates then displays the result of the division numerator/denominator. Make sure to display a message stating that division by zero is an illegal operation if the denominator is zero.
Exercise 8:
Write a Python program that receives the current temperature as input. If the temperature is 30 degrees or more, output a message telling the user to go swimming. Otherwise, if the temperature is 15 degrees or more, output a message to go running. Otherwise, stay inside.
Exercise 9:
Your health status depends on your Body Mass Index (BMI). BMI is a person’s weight in kilograms or pounds divided by the square of height in meters or inches. The formula for BMI is weight in kilograms divided by height in meters squared. If height has been measured in centimeters, divide by 100 to convert this to meters. See below the BMI ranges. When using English measurements, pounds should be divided by inches squared. This should then be multiplied by 703 to convert from pounds per square inch to kg/m^2.
Write a Python program that reads in your weight and your height, then displays your health status: Underweight, Healthy, Overweight, or Obese. Your program should accept both metric (kg, cm) or English units (lb, inches). Your program should test the validity of the input, that is, both weight and height are positive.
BMI ranges:
– Below 18.5: Underweight
– 18.5-24.9: Healthy
– 25.0-29.9: Overweight
– 30.0 or higher: Obese.
