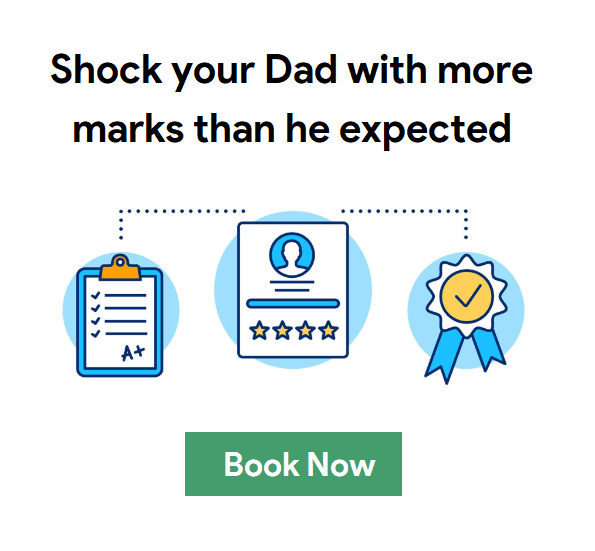
Create JAVA program to model a simple part of banking system. You will have three classes: Balance, Bank, and Transaction. ⚫ Create a Balance class: ⚫ With private attributes: 1. balance (double) 2. accountNo (int) 3. date (Date) [using Date class as in the lectures] ⚫ With the following methods 1. No-argument constructor 2. User define constructors 3. Getter and setter methods for all attributes. 4. Override the method toString ⚫ Create a TransactionType enum: Withdraw, Deposite, and ShowInfo ⚫ Create a Bank class: ⚫ With private attributes: BlanceList (Array List of type Balance) ⚫ With the following methods 1. No-argument constructor 2. User define constructors 3. addAccount: this method should add a balance to the defined array list. 4. searchAccount: this method should search the account and return it. 5. removeAccount [as variable length argument lists]: this method should remove the whole account from the defined array list. 6. getBalanceListSize 7. Override the method toString ⚫ Create Transaction class: ⚫ With private attributes: 1. TansactionType (enum) 2. amount (double) 3. accountNo (int) 4. date (Date) 5. bank (Bank) 6. balance (Balance) 7. overlimit (double): the maximum amount you can withdraw ⚫ With the following methods 1. No-argument constructor 2. User define constructors 3. transactOperation • Deposit: to deposit money into the account. • Withdraw: to withdraw money from the account. • Show Info: show information about the balance 4. Override the method toString ⚫ Notes: You should handle all error cases with Dialog Box: 1. The accountNo does not exist. 2. Withdraw amount > balance 3. Withdraw amount> overLimit Do not forget memory management (Garbage Collector)
